A fractal is a geometric object that has self-similarity — it shows the same pattern whether you’re looking at it far away or close up. Fractals can provide mathematically accurate models of coastlines, mountains, and other real objects from nature. Loops (specifically, recursive loops) are used to code fractals. One of the earliest uses of computer graphics in big-screen movies was the creation of fractal-based scenery for the transformation of the Genesis planet in Star Trek II: The Wrath of Khan. Khhannnnnn!
Using pseudocode
Nesting loops can happen in any combination. Here are some examples of nesting loops, but this list is not exhaustive.For-each loop nested inside a for-each loop:
for(item in list) action1 for(item in list) action2 action3 … END_FOR_EACH action4 END_FOR_EACHExample:
jellyBeanCount = 0 for(jar in jars) for(jellybean in jar) jellyBeanCount = jellyBeanCount + 1 END_FOR_EACH END_FOR_EACHWhile loop nested inside a for-each loop:
for(item in list) action1 while(condition) action2 action3 … END_WHILE action4 END_FOR_EACHExample:
for(room in house) while(wallsWhite) paintWallsBlue END_WHILE END_FOR_EACH
Using Scratch
Nesting loops in Scratch can be a lot of fun when you introduce the pen and start drawing fun shapes. Here are some basic examples of nesting loops in Scratch.Repeat loop nested inside a repeat loop
The image below shows an example of a Scratch program that draws 10 concentric squares. The outer repeat loop is responsible for repeating 10 times for each square. The inner repeat loop is responsible for repeating 4 times, for each line and turn in each square.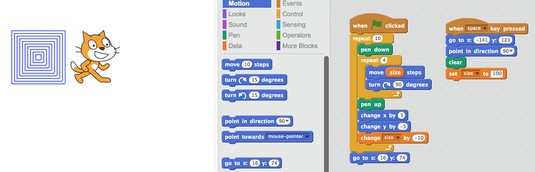
Repeat loop nested inside a repeat-until loop
Take a look at the image below to see an example of a Scratch program that draws squares in random places around the screen until the user presses the space key.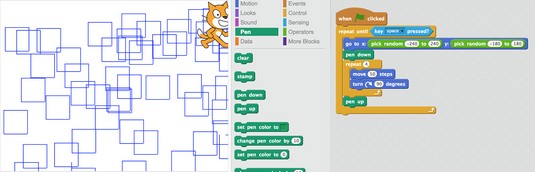
Using Python
One of the most fun programs to write in Python with nested loops is ASCII art. In coding, each character and symbol that you can type on a keyboard has a number representation called its ASCII number. Although in today’s programming languages, you can type the character or symbol in your program, in the past you would have to use the ASCII number representation. ASCII art is basically when you create a picture using characters or symbols. There are some pretty amazing ASCII art examples. As a kid, Camille used to have ASCII portraits of all the Star Trek: The Original Series cast members on her wall!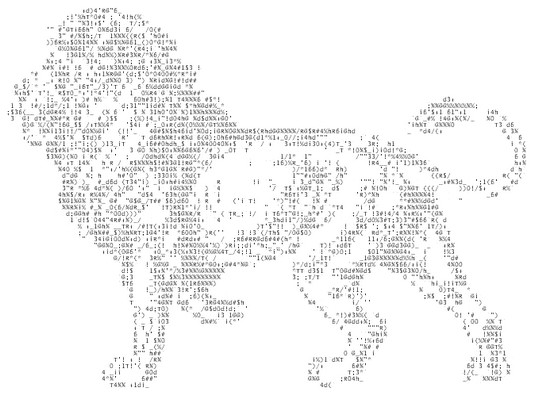
You can create a simple ASCII art program in Python using nested loops! For example, to create this pattern:
# ## ### #### ##### ###### ####### ######## #########The algorithm might be:
Print 1 # on Row 1 Print 2 # on Row 2 Print 3 # on Row 3 …You could represent this using nested loops too!
for row in range(1, 11): rowText = '' for column in range(1, row): rowText = rowText + '#' print rowText</pre